Introduction
In Python programming, the meticulous art of output formatting assumes significance beyond syntax. This article is not just a technical walkthrough; it’s a reminder that how we present information is inseparable from the code itself. The precision embedded in formatting is not a matter of aesthetics but a silent guide, reducing ambiguity, enhancing readability, and elevating the user experience. As we explore Python’s output formatting techniques, let’s recognize that our commitment to precision is not a mere stylistic choice but a fundamental aspect of crafting code that withstands scrutiny and stands the test of time.
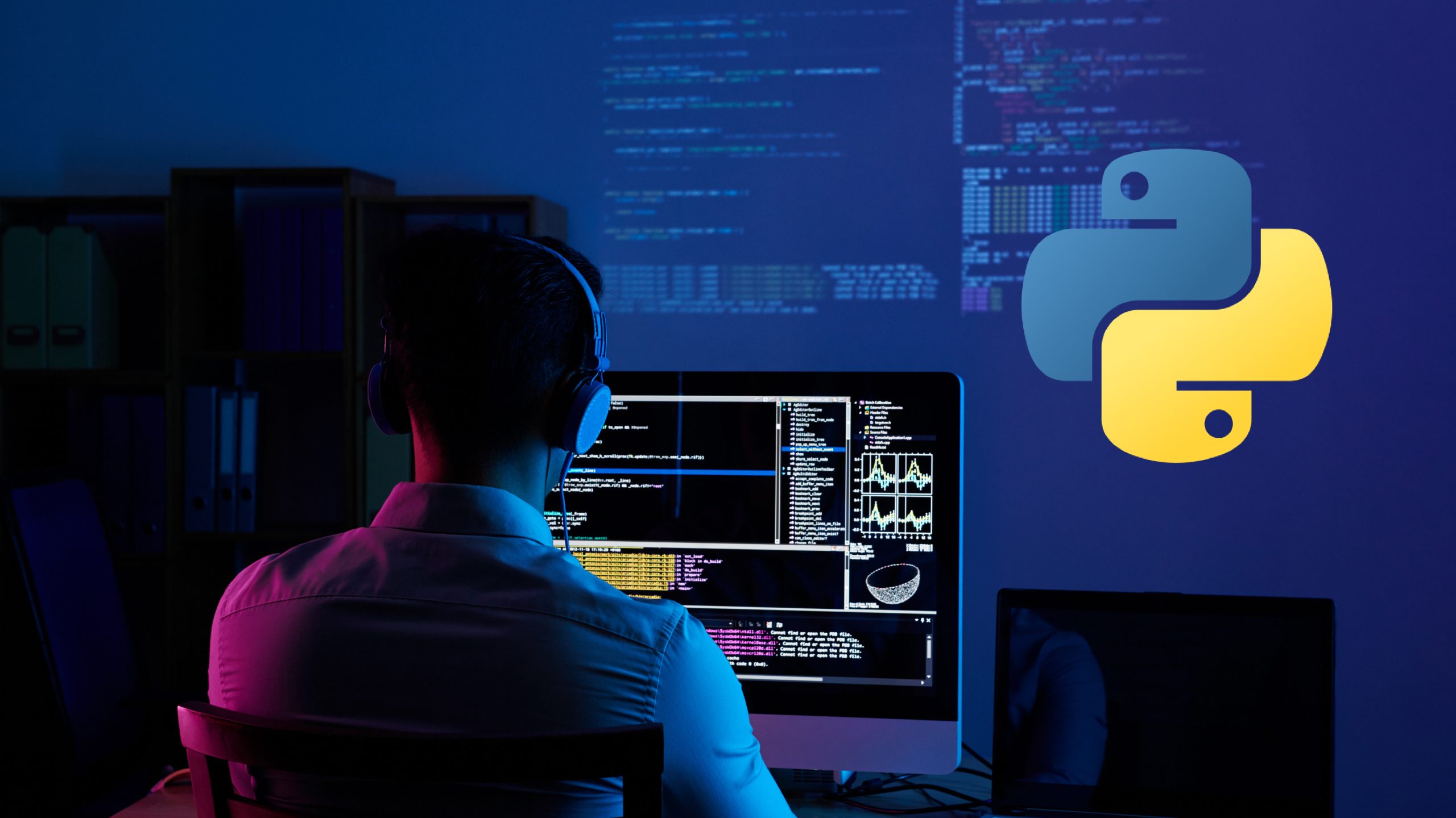
Why is Output Formatting important?
Proper output formatting plays a crucial role in programming. It improves the readability of the code and enhances the user experience. Well-formatted output makes it easier for users to understand the information presented and reduces the chances of misinterpretation. Additionally, formatted output adds a professional touch to your code and makes it more presentable.
Basic Output Formatting Techniques
Using the print() Function
The print() function is the most basic way to display output in Python. It allows you to print strings, variables, and expressions. For example:
Code
name = "John"
age = 25
print("My name is", name, "and I am", age, "years old.")
Output
My name is John and I am 25 years old.
Formatting Strings with Placeholders
Python provides placeholders that allow you to insert values into strings dynamically. The `%` operator is used for string formatting. For example:
Code
name = "John"
age = 25
print("My name is %s and I am %d years old." % (name, age))
Output
My name is John and I am 25 years old.
Concatenating Strings for Output
Another way to format output is by concatenating strings using the `+` operator. This method allows you to combine multiple strings into a single output. For example:
Code
name = "John"
age = 25
print("My name is " + name + " and I am " + str(age) + " years old.")
Output
My name is John and I am 25 years old.
Formatting Numeric Output
Formatting Integers
To format integers, you can use the `%d` placeholder. It allows you to specify the width and precision of the output. For example:
Code
num = 12345
print("The number is %10d." % num)
Output
The number is 12345.
Formatting Floating-Point Numbers
You can use the `%f` placeholder to format floating-point numbers. It allows you to control the width and precision of the output. For example:
Code
pi = 3.14159
print("The value of pi is %.2f." % pi)
Output
The value of pi is 3.14.
Formatting Decimal Numbers
You can use the `%g` placeholder to format decimal numbers. It automatically adjusts the precision based on the value. For example:
Code
num = 123.456789
print("The number is %g." % num)
Output
The number is 123.457.
Formatting Scientific Notation
Python provides the `%e` placeholder for formatting numbers in scientific notation. It allows you to control the width and precision of the output. For example:
Code
num = 123456789
print("The number is %.2e." % num)
Output
The number is 1.23e+08.
Formatting Text Output
Aligning Text
To align text, you can use the `ljust()`, `rjust()`, or `center()` methods. These methods allow you to specify the width and fill characters for alignment. For example:
Code
text = "Hello"
print(text.ljust(10, "-"))
print(text.rjust(10, "-"))
print(text.center(10, "-"))
Output
Hello—–
—–Hello
–Hello—
Controlling Width and Precision
Python provides various methods to control the width and precision of text output. The `format()` method allows you to specify the width, precision, and alignment. For example:
Code
name = "John"
age = 25
print("My name is {:10} and I am {:<5} years old.".format(name, age))
Output
My name is John and I am 25 years old.
Formatting Dates and Times
You can use the `strftime()` method to format dates and times. It allows you to specify the format codes for different date and time components. For example:
Code
import datetime
now = datetime.datetime.now()
print(now.strftime("Today is %A, %B %d, %Y. The time is %I:%M %p."))
Output
Today is Monday, October 18, 2021. The time is 09:30 AM.
Formatting Currency
Python provides the `locale` module for formatting currency values. It allows you to format currency based on the user’s locale settings. For example:
Code
import locale
locale.setlocale(locale.LC_ALL, 'en_US.UTF-8')
amount = 1234.56
print(locale.currency(amount))
Output
$1,234.56
Also read: How to Use PPrint in Python? [Explained with Examples]
Advanced Output Formatting Techniques
Using Format Specifiers
Format specifiers provide a more flexible way to format output in Python. They allow you to specify the format using curly braces `{}` and provide additional options. For example:
Code
name = "John"
age = 25
print("My name is {} and I am {} years old.".format(name, age))
Output
My name is John and I am 25 years old.
Using f-strings
f-strings are a more recent addition to Python and provide a concise way to format output. They allow you to embed expressions directly into strings using curly braces `{}`. For example:
Code
name = "John"
age = 25
print(f"My name is {name} and I am {age} years old.")
Output
My name is John and I am 25 years old.
Formatting Output with Templates
Python provides the `string.Template` class for advanced output formatting. It allows you to define templates with placeholders and substitute values dynamically. For example:
Code
from string import Template
template = Template("My name is $name and I am $age years old.")
print(template.substitute(name=name, age=age))
Output
My name is John and I am 25 years old.
Customizing Output with Format Options
Python allows you to customize output further by using format options. These options include specifying the width, precision, alignment, and fill character. For example:
Code
name = "John"
age = 25
print(f"My name is {name:10} and I am {age:<5} years old.")
Output
My name is John and I am 25 years old.
Handling Special Characters and Escape Sequences
Escaping Characters
To include special characters or escape sequences in strings, you can use the backslash `\`. It allows you to include characters like quotes, newlines, tabs, etc. For example:
Code
print("She said, \"Hello!\"")
Output
She said, “Hello!”
Handling Newlines and Tabs
Python provides escape sequences like `\n` for newlines and `\t` for tabs. These sequences allow you to control the formatting of text output. For example:
Code
print("Line 1\nLine 2\nLine 3")
print("Column 1\tColumn 2\tColumn 3")
Output
Line 1
Line 2
Line 3
Column 1 Column 2 Column 3
Dealing with Unicode Characters
Python supports Unicode characters, allowing you to include special characters from different languages and symbol sets. You can use Unicode escape sequences or directly include the characters in strings. For example:
Code
print("Hello, \u03A9!") # Unicode escape sequence
print("Currency symbol: \u20AC") # Euro symbol
Output
Hello, Ω!
Currency symbol: €
Conclusion
Proper output formatting is crucial for effectively presenting information in Python. In this comprehensive guide, we explored various techniques for formatting output. From basic techniques like using the print() function to advanced methods like f-strings and format specifiers, Python offers a wide range of options for formatting output. By following these techniques, you can enhance the readability and professionalism of your code. So, apply these formatting techniques in your Python projects and make your output shine!