Introduction
When working with lists in Python, finding the average values within the list is often necessary. The average, also known as the arithmetic mean, is a measure of central tendency that provides insight into the overall value of a dataset. In this article, we will explore 7 methods for finding the average list in Python, along with examples and tips for handling different scenarios.
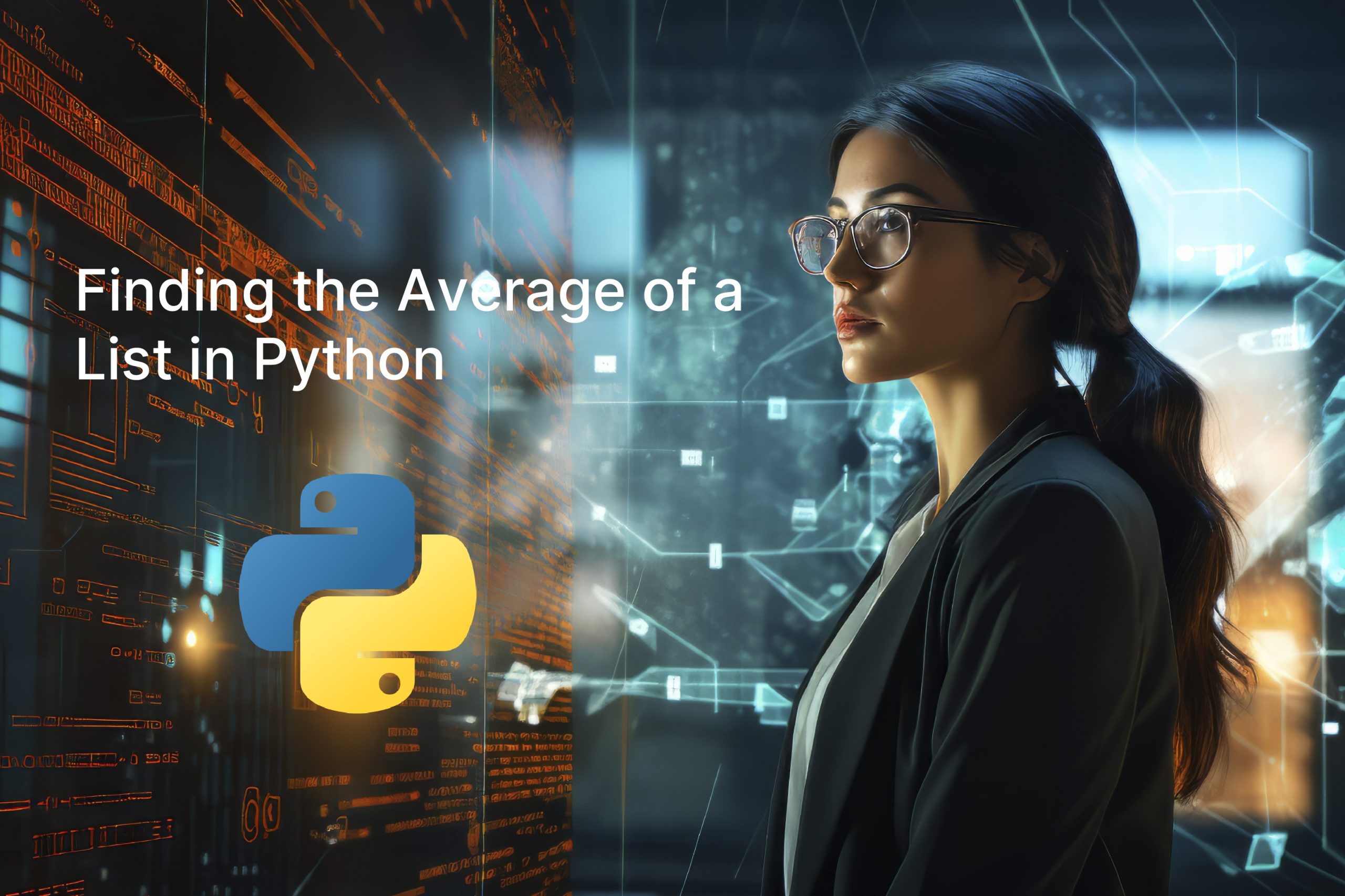
Methods for Finding the Average in Python
Using a For Loop
One of Python’s simplest methods for finding a list’s average is a for loop. This method involves iterating over each element in the list, summing up the values, and then dividing the sum by the length of the list. Here’s an example:
Code
def average_with_for_loop(lst):
total = 0
for num in lst:
total += num
average = total / len(lst)
return average
Utilizing the sum() and len() Functions
Python provides built-in functions like `sum()` and `len()` that can be used to simplify finding the average of a list. Using these functions, we can calculate the sum of the list and divide it by the list length to obtain the average. Here’s an example:
Code
def average_with_sum_len(lst):
average = sum(lst) / len(lst)
return average
Implementing the Statistics Module
Python’s `statistics` module offers a convenient way to find the average of a list. The `mean()` function from this module can be used to calculate the arithmetic mean of a list. Here’s an example:
Code
import statistics
def average_with_statistics(lst):
average = statistics.mean(lst)
return average
Using the numpy Library
The `numpy` library is a powerful tool for scientific computing in Python. It provides a wide range of functions, including finding the average of a list. Using the `numpy.mean()` function, we can easily calculate the average of a list. Here’s an example:
Code
import numpy as np
def average_with_numpy(lst):
average = np.mean(lst)
return average
Creating a Custom Function
In some cases, you may need to customize the process of finding the average based on specific requirements. By creating a custom function, you can implement your own logic for calculating the average of a list. Here’s an example:
Code
def custom_average(lst):
total = 0
count = 0
for num in lst:
if num % 2 == 0:
total += num
count += 1
average = total / count
return average
Examples of Finding the Average in Python
Example 1: Finding the Average of Integers
Let’s say we have a list of integers, and we want to find the average. We can use any of the methods mentioned above to calculate the average. Here’s an example using the `average_with_sum_len()` function:
Code
numbers = [1, 2, 3, 4, 5]
average = average_with_sum_len(numbers)
print("The average of the list is:", average)
Output
The average of the list is: 3.0
Example 2: Finding the Average of Floating-Point Numbers
If the list contains floating-point numbers, the methods mentioned earlier can still be used to find the average. Here’s an example using the `average_with_for_loop()` function:
Code
numbers = [1.5, 2.5, 3.5, 4.5, 5.5]
average = average_with_for_loop(numbers)
print("The average of the list is:", average)
Output
The average of the list is: 3.5
Example 3: Finding the Average of a List with Mixed Data Types
In some cases, the list may contain mixed data types, such as integers and floating-point numbers. The methods mentioned earlier can handle such scenarios as well. Here’s an example using the `average_with_statistics()` function:
Code
data = [1, 2.5, 3, 4.5, 5]
average = average_with_statistics(data)
print("The average of the list is:", average)
Output
The average of the list is: 3.0
Also read: 15 Essential Python List Functions & How to Use Them (Updated 2024)
Tips and Tricks for Finding the Average in Python
Handling Empty Lists
When dealing with empty lists, it is important to handle them gracefully to avoid errors. One way to handle empty lists is by checking if the list is empty before calculating the average. Here’s an example:
Code
def average_with_empty_list(lst):
if len(lst) == 0:
return 0
average = sum(lst) / len(lst)
return average
Dealing with Large Lists
If you are working with large lists, it is important to consider the performance of your code. Using methods like `numpy.mean()` or `statistics.mean()` can be more efficient for large datasets than a for loop. These methods are optimized for performance and can handle large lists efficiently.
Rounding the Average
In some cases, you may want to round the average to a specific number of decimal places. Python provides the `round()` function to round the average to the desired precision. Here’s an example:
Code
average = 3.14159
rounded_average = round(average, 2)
print("The rounded average is:", rounded_average)
Output
The rounded average is: 3.14
Handling Errors and Exceptions
When working with user input or external data, it is important to handle errors and exceptions. For example, if the list contains non-numeric values, an error may occur during calculating the average. By using try-except blocks, you can catch and handle these errors gracefully. Here’s an example:
Code
def average_with_error_handling(lst):
try:
average = sum(lst) / len(lst)
return average
except ZeroDivisionError:
return 0
except TypeError:
return "Invalid data type in the list"
Optimizing Performance
If you need to find the average of a list multiple times, it is more efficient to calculate it once and store the result for future use. This can help optimize the performance of your code, especially when dealing with large datasets.
Conclusion
Finding the average of a list is a common task in Python programming. In this article, we explored various methods for calculating the average, including using for loops, built-in functions, modules, libraries, and custom functions. We also provided examples and tips for handling different scenarios, such as empty lists, large lists, roundiAverage of a List in Pythonng, error handling, and performance optimization. By understanding these techniques, you can effectively find the average of a list in Python and apply it to your projects.
If you are looking for a Python course online, explore – Learn Python for Data Science.