Introduction
Converting a string to a list is a common task in Python programming. It allows us to manipulate and work with individual elements of a string more easily. One popular method for converting a string to a list in Python is using the list() constructor. This constructor takes an iterable, such as a string, and converts it into a list by considering each character as a separate element. In this article, we will explore different methods to convert a string to a list in Python. We will also provide examples and code snippets to demonstrate each method.
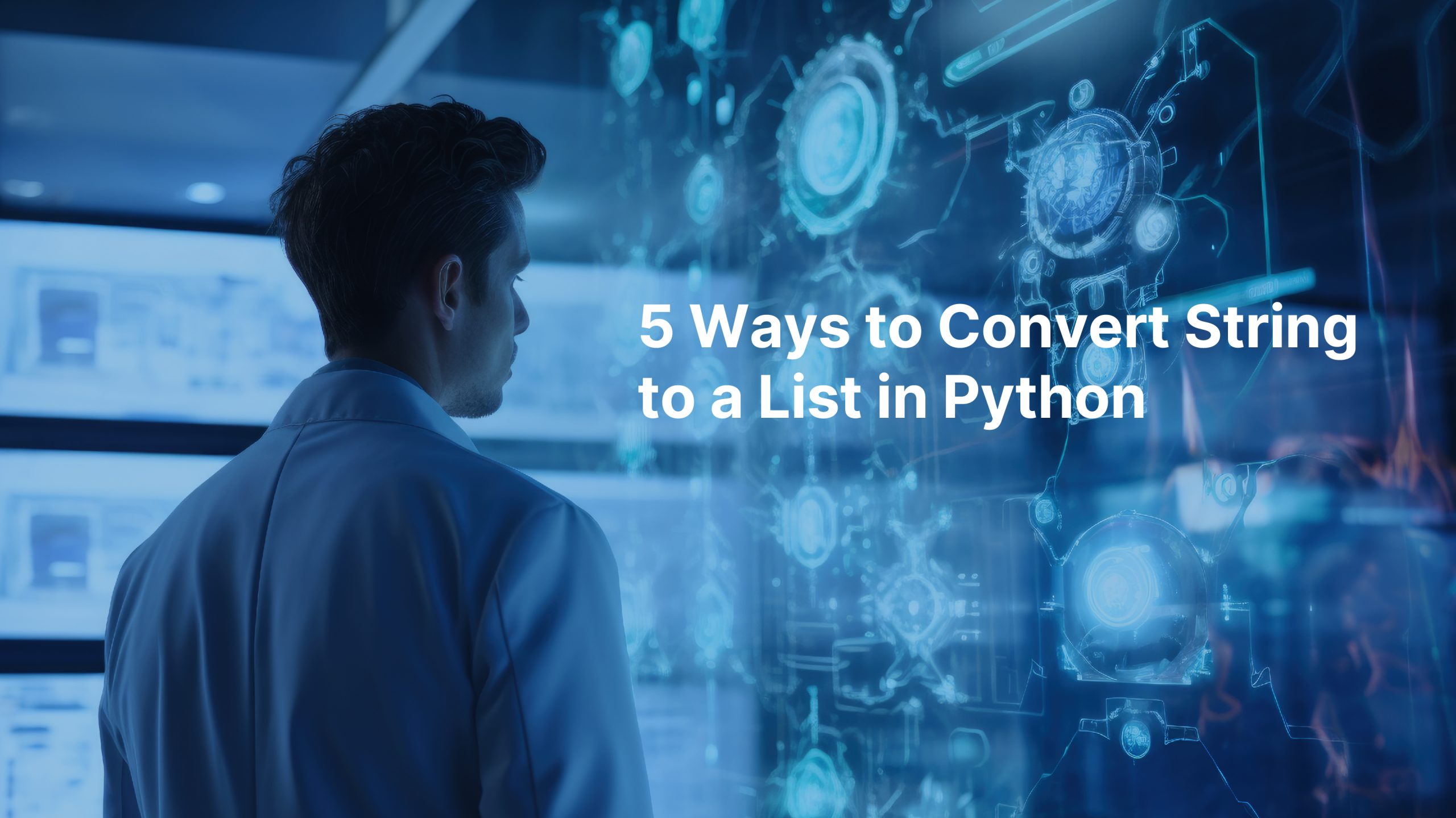
Methods to Convert String to a List in Python
Using the split() Method
The split() method is a built-in function in Python that splits a string into a list of substrings based on a specified delimiter. By default, the delimiter is a space. Here’s an example:
string = "Hello World"
list = string.split()
print(list)
Output
[‘Hello’, ‘World’]
Using List Comprehension
List comprehension is a concise way to create lists in Python. It allows us to iterate over a string and append each character to a list. Here’s an example:
string = "Hello World"
list = [char for char in string]
print(list)
Output
[‘H’, ‘e’, ‘l’, ‘l’, ‘o’, ‘ ‘, ‘W’, ‘o’, ‘r’, ‘l’, ‘d’]
Using the map() Function
The map() function applies a given function to each item in an iterable and returns a new list. We can use the map() function and the list() function to convert a string to a list. Here’s an example:
string = "Hello World"
list = list(map(str, string))
print(list)
Output
[‘H’, ‘e’, ‘l’, ‘l’, ‘o’, ‘ ‘, ‘W’, ‘o’, ‘r’, ‘l’, ‘d’]
Using the ast.literal_eval() Function
The ast.literal_eval() function evaluates a string containing a Python literal or container and returns the corresponding Python object. We can use this function to convert a string to a list. Here’s an example:
import ast
string = "[1, 2, 3, 4, 5]"
list = ast.literal_eval(string)
print(list)
Output
[1, 2, 3, 4, 5]
Using Regular Expressions
Regular expressions provide a powerful way to manipulate strings in Python. We can use the re.split() function from the re module to split a string into a list based on a regular expression pattern. Here’s an example:
import re
string = "Hello,World"
list = re.split(r',', string)
print(list)
Output
[‘Hello’, ‘World’]’
Also read: 5 Ways of Finding the Average of a List in Python
Examples of String to List Conversion
Converting a Comma-Separated String to a List
string = "apple,banana,orange"
list = string.split(',')
print(list)
Output
[‘apple’, ‘banana’, ‘orange’]
Converting a String with Nested Elements to a List
import ast
string = "[1, [2, 3], [4, [5, 6]]]"
list = ast.literal_eval(string)
print(list)
Output
[1, [2, 3], [4, [5, 6]]]
Tips for Efficient String to List Conversion in Python
Handling Empty Strings
Converting an empty string to a list will result in an empty list. It is important to handle this case to avoid any unexpected errors in your code.
Dealing with Whitespace and Punctuation
When using the split() method or regular expressions to split a string into a list, be aware of leading or trailing whitespace and punctuation marks. These can affect the resulting list.
Handling Error Cases
When using the ast.literal_eval() function, ensure the string contains a valid Python literal or container. Otherwise, it may raise a ValueError.
Conclusion
Converting a string to a list is a fundamental operation in Python programming. In this article, we explored various methods to achieve this conversion, including using the split() method, list comprehension, the map() function, ast.literal_eval(), and regular expressions. We also provided examples and code snippets to demonstrate each method. By understanding these techniques, you can efficiently convert strings to lists and manipulate them as needed in your Python programs.
If you are looking for a Python course online, explore – Learn Python for Data Science.