Introduction
Lists, one of the built-in data types in Python, play a crucial role in storing and managing collections of items. When working with lists, iterating over their elements is a common task. This process of accessing each element in a list is known as iteration. In this article, we will explore the various methods and techniques to iterate over a list in Python, along with their benefits and use cases.
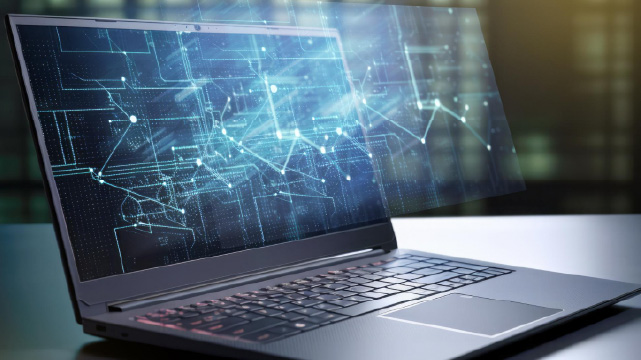
What is Iteration in Python?
Iteration is accessing each element in a collection, such as a list and performing a specific operation. It allows us to iterate over the elements of a list one by one, enabling us to manipulate, analyze, or extract information from the list.
Benefits of Iterating over a List
Iterating over a list provides several benefits, including:
Flexibility
Iteration allows us to perform various operations on each list element, such as accessing, modifying, searching, filtering, or performing mathematical operations.
Efficiency
We can process each element individually by iterating over a list, which can be more efficient than manually performing the same operation on each element.
Readability
Iteration makes the code more readable and understandable, indicating that a specific operation is being performed on each list element.
You can also read: All You Need to Know About Python Lists
Different Methods to Iterate over a List
There are several methods available in Python to iterate over a list. Let’s explore each of them in detail:
Using a For Loop
A for loop is the most common and straightforward method to iterate over a list. The for loop lets us iterate over each list element and perform a specific operation. Here’s an example:
fruits = ["apple", "banana", "orange"]
for fruit in fruits:
print(fruit)
Output
apple
banana
orange
Using a While Loop
Another method to iterate over a list is by using a while loop. The while loop allows us to iterate over the list until a specific condition is met. Here’s an example:
fruits = ["apple", "banana", "orange"]
index = 0
while index < len(fruits):
print(fruits[index])
index += 1
Output
apple
banana
orange
Using List Comprehension
List comprehension is a concise and powerful way to iterate over a list and perform operations on its elements in a single line of code. It allows us to create a new list based on the existing list. Here’s an example:
fruits = ["apple", "banana", "orange"]
uppercased_fruits = [fruit.upper() for fruit in fruits]
print(uppercased_fruits)
Output
[‘APPLE’, ‘BANANA’, ‘ORANGE’]
Using the enumerate() Function
The enumerate() function is used to iterate over a list while keeping track of each element’s index. It returns a tuple containing the index and the element itself. Here’s an example:
fruits = ["apple", "banana", "orange"]
for index, fruit in enumerate(fruits):
print(index, fruit)
Output
0 apple
1 banana
2 orange
Using the zip() Function
The zip() function allows us to iterate over multiple lists simultaneously. It combines the elements from each list into tuples and returns an iterator. Here’s an example:
fruits = ["apple", "banana", "orange"]
colors = ["red", "yellow", "orange"]
for fruit, color in zip(fruits, colors):
print(fruit, color)
Output
apple red
banana yellow
orange orange
Using the itertools Module
The itertools module provides various functions for efficient iteration. One such function is the itertools.cycle() function allows us to iterate over a list indefinitely. Here’s an example:
import itertools
fruits = ["apple", "banana", "orange"]
for fruit in itertools.cycle(fruits):
print(fruit)
Output
apple
banana
orange
apple
banana
orange
Common Use Cases for Iterating over a List
Iterating over a list is useful in various scenarios. Let’s explore some common use cases:
Accessing List Elements
We can access each element individually by iterating over a list and performing specific operations on them.
Modifying List Elements
We can iterate over a list and modify its elements based on certain conditions. For example, let’s double each number in a list:
numbers = [1, 2, 3, 4, 5]
for i in range(len(numbers)):
numbers[i] *= 2
print(numbers)
Output
[2, 4, 6, 8, 10]
Searching for Specific Values
By iterating over a list, we can search for specific values and perform actions based on their presence or absence. For example, let’s check if a list contains a specific number:
numbers = [1, 2, 3, 4, 5]
target = 3
for number in numbers:
if number == target:
print("Number found!")
break
else:
print("Number not found!")
Output
Number found!
Filtering List Elements
We can iterate over a list and filter out elements based on certain conditions. For example, let’s filter out even numbers from a list:
numbers = [1, 2, 3, 4, 5]
filtered_numbers = [number for number in numbers if number % 2 == 0]
print(filtered_numbers)
Output
[2, 4]
Performing Mathematical Operations
We can perform mathematical operations on its elements by iterating over a list. For example, let’s calculate the sum of all numbers in a list:
numbers = [1, 2, 3, 4, 5]
sum = 0
for number in numbers:
sum += number
print(sum)
Output
15
Tips and Tricks for Efficient List Iteration
To make list iteration more efficient and effective, consider the following tips and tricks:
Avoiding Unnecessary Iterations
Avoid unnecessary iterations by breaking out of loops or using conditional statements to skip certain elements. This can improve the performance of your code.
Using List Slicing
List slicing allows you to iterate over a specific subset of a list. It can be useful when you only need to iterate over a portion of the list.
Leveraging Built-in Functions and Methods
Python provides several built-in functions and methods that can simplify list iteration. For example, the sum() function can be used to calculate the sum of all elements in a list.
Exploring Alternative Iteration Techniques
Python offers various libraries and modules that provide alternative iteration techniques. For specialized iteration requirements, explore libraries like NumPy or pandas.
Conclusion
Iterating over a list is a fundamental operation in Python. Remember to follow best practices and consider performance trade-offs when writing iteration code. You can effectively manipulate, analyze, and extract information from lists by understanding the different methods and techniques available for list iteration.