Introduction
Variables are an essential part of any programming language, including Python. They allow us to store and manipulate data within our programs. In Python, variables can have different scopes, which determine their accessibility and visibility within the program. In this article, we will explore the concepts of global and local variables in Python, understand their differences, and discuss best practices for using them effectively.
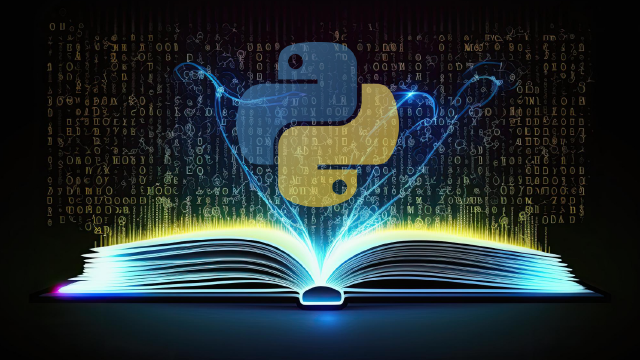
What are Variables in Python?
Variables in Python are used to store data values that can be accessed and manipulated throughout the program. They act as containers for holding different types of data, such as numbers, strings, or objects. In Python, variables are dynamically typed, meaning that their type can change during runtime.
Understanding Scope in Python
Scope refers to the region of a program where a variable is defined and can be accessed. In Python, there are three types of variable scopes: global, local, and nonlocal.
Global Variables
Global variables are defined outside any function or class and can be accessed from anywhere within the program. They have a global scope, meaning they are visible to all functions and classes. Global variables are useful when you want to share data between different parts of your program.
Local Variables
Local variables are defined within a function or a block of code and can only be accessed within that specific function or block. They have a local scope, meaning they are only visible within the function or block where they are defined. Local variables are temporary and are destroyed once the function or block of code is executed.
Nonlocal Variables
Nonlocal variables are used in nested functions, where a function is defined inside another function. They are neither global nor local variables. Nonlocal variables can be accessed and modified by the inner function, as well as the outer function that encloses it.
Examples and Use Cases of Global and Local Variables in Python
Let’s explore some examples and use cases to understand the practical applications of global and local variables in Python.
Global Variables in Python
count = 0
def increment():
global count
count += 1
increment()
print(count)
Output
1
In this example, we have a global variable `count` that is incremented inside the `increment()` function using the `global` keyword. The updated value of `count` is then printed, which is 1.
Local Variables in Python
def calculate_sum(a, b):
result = a + b
return result
sum = calculate_sum(5, 10)
print(sum)
Output
15
In this example, we have a local variable `result` that is defined within the `calculate_sum()` function. The function takes two arguments, `a` and `b`, and calculates their sum. The result is stored in the `result` variable, which is then returned and assigned to the global variable `sum`. Finally, the value of `sum` is printed, which is 15.
Nonlocal Variables in Python
def outer_function():
x = 10
def inner_function():
nonlocal x
x += 5
print(x)
# Output: 15
inner_function()
outer_function()
In this example, we have a nonlocal variable `x` that is defined in the `outer_function()`. The `inner_function()` is a nested function that accesses and modifies the value of `x` using the `nonlocal` keyword. The updated value of `x` is then printed, which is 15.
Differences Between Global and Local Variables in Python
There are several differences between global and local variables in Python, including their definition and declaration, access and visibility, lifetime and memory allocation, and modifiability and immutability.
Definition and Declaration
Global variables are declared outside any function or class, while local variables are declared within a function or block of code. Global variables can be accessed from anywhere in the program, whereas local variables are only accessible within the function or block where they are defined.
Access and Visibility
Global variables have a wider scope and can be accessed from any part of the program. Local variables, on the other hand, have a limited scope and can only be accessed within the function or block where they are defined.
Lifetime and Memory Allocation
Global variables have a longer lifetime as they are created when the program starts and exist until the program terminates. They are stored in a separate memory location. Local variables, on the other hand, have a shorter lifetime and are created when the function or block of code is executed. They are stored in the stack memory.
Modifiability and Immutability
Global variables can be modified throughout the program, and their values can be changed multiple times. Local variables, however, are temporary and can only be modified within the function or block where they are defined.
Best Practices for Using Global and Local Variables in Python
To ensure clean and maintainable code, it is important to follow some best practices when using global and local variables in Python.
Avoiding Variable Name Conflicts
When using global and local variables, it is crucial to choose variable names that are unique and do not conflict with each other. This helps in avoiding unexpected behavior and makes the code more readable.
Using Global Variables Sparingly:
Global variables should be used sparingly as they can make the code harder to understand and debug. It is recommended to encapsulate code in functions and use local variables whenever possible.
Encapsulating Code in Functions
Encapsulating code in functions promotes code reusability and modularity. It allows for better organization of variables and reduces the chances of naming conflicts.
Using Nonlocal Variables in Nested Functions
When working with nested functions, nonlocal variables can be used to access and modify variables from the outer function. This provides a way to share data between nested functions without using global variables.
Advanced Topics and Techniques
In addition to global and local variables in Python, there are some advanced topics and techniques related to variable scope in Python.
Global Variables in Modules
Global variables can be defined within modules and accessed from different parts of the program. This allows for sharing data between multiple files and promotes code reusability.
Global Variables in Classes
In object-oriented programming, global variables can be defined within classes and accessed by different methods of the class. This provides a way to store and share data across different instances of the class.
Dynamic Variable Scoping
Python supports dynamic variable scoping, which means that the scope of a variable can change during runtime. This allows for more flexibility in accessing and modifying variables based on the program’s logic.
Variable Scope in Nested Functions
Nested functions in Python have access to variables from the outer function’s scope. This allows for data sharing and encapsulation of code within nested functions.
Conclusion
Understanding the concepts of global and local variables in Python is crucial for writing efficient and maintainable code. By following the best practices and avoiding common mistakes, you can effectively use global and local variables to store and manipulate data within your programs. Remember to choose variable names wisely, encapsulate code in functions, and use global variables sparingly. With a solid understanding of variable scope in Python, you can write cleaner and more organized code.
Ready to deepen your Python skills and embark on an exciting journey into the realms of Artificial Intelligence and Machine Learning? Enroll in our Certified AI & ML BlackBelt Plus Program and take your Python proficiency to the next level. Gain hands-on experience, unlock advanced concepts, and position yourself as an AI and ML expert. Don’t miss this opportunity to become a certified Python BlackBelt—sign up now and elevate your programming prowess! Learn more about Python by enrolling in the Certified AI & ML BlackBelt Plus Program today.