Introduction
In the world of Python programming, modularity is key. It’s common to segment your code into different modules and directories for better organization and reusability. But what happens when you need to import a module from a directory that isn’t the current one? This can be a head-scratcher for many developers. Fear not! In this blog post, we’ll dive into the various methods to import modules from different directories, ensuring your code remains clean and efficient.
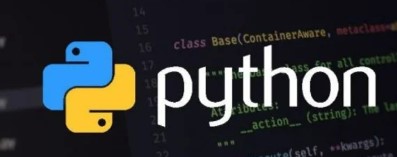
Understanding Python’s Import System
Before we start bending Python’s import system to our will, it’s crucial to understand how it works. Python’s import statement is more than just a way to use code from another file; it’s a gateway to a vast ecosystem of libraries and modules. When you import a module, Python searches through a list of directories defined in sys.path. By default, this list includes the directory containing the input script (or the current directory), along with the standard library directories. Understanding this search path is the first step in mastering module imports from different directories.
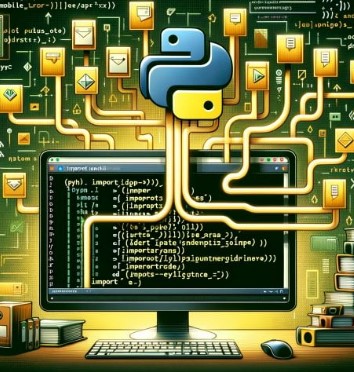
Modifying sys.path
One straightforward way to import a module from a different directory is by appending the module’s directory to sys.path. This method is simple and doesn’t require any structural changes to your project. However, it’s considered a temporary solution and can lead to code that’s harder to maintain. Here’s how you can do it:
import sys
sys.path.append('/path/to/your/module/directory')
import your_module
Using this snippet, Python will now include the specified directory when searching for modules to import.
Understanding PYTHONPATH’s Influence
The PYTHONPATH environment variable is a powerful tool for influencing Python’s module and package search behavior. By configuring PYTHONPATH, you can specify additional directories where Python should look for modules and packages. Unlike directly modifying sys.path within your code, setting PYTHONPATH occurs externally to Python. Typically, you configure PYTHONPATH in your shell’s configuration file or through your operating system’s environment settings. This approach allows you to customize the module search path without altering the code, providing a flexible and environment-based solution.
To define the path in Linux via the terminal, utilize the command:
export PYTHONPATH='/path/to/your/module/directory’
For Windows systems, employ the following command:
SET PYTHONPATH=”path/to/directory”
Understanding Relative Imports
In Python, relative imports enable you to import modules by specifying their location relative to the current module within the directory structure. This is particularly useful when working within a package, as you can utilize dot notation to traverse the hierarchy. While effective in such contexts, it’s important to note that relative imports come with limitations and may not be the ideal choice for every scenario, particularly when working with standalone scripts outside of a package.
Consider a directory structure like this:
project/
|– package/
| |– __init__.py
| |– module1.py
| |– module2.py
|
|– script.py
To run script.py, you would execute it from the project directory. This example showcases how relative imports can be used within a package structure to organize and access modules. However, keep in mind the limitations mentioned, particularly when dealing with standalone scripts outside a package.
Creating a Package
If you often find yourself requiring modules from diverse directories, it’s worth considering the creation of a package to organize your code. In Python, a package is essentially a directory containing a special file named init.py (which can be left empty) and can accommodate both modules and sub-packages. Structuring your code in this way enables the use of both absolute and relative imports, providing an efficient approach to managing your modules.
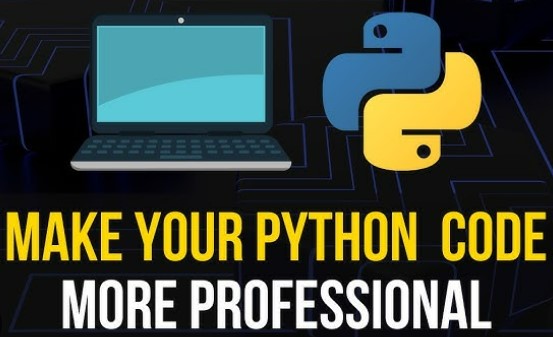
Enroll in our free Python Course today.
Consider the following directory structure:
my_package/
|– __init__.py
|– module1.py
|– module2.py
|– subpackage/
| |– __init__.py
| |– module3.py
|– script.py
module1.py:
# module1.py
def greet():
return "Hello from module1!"
module2.py:
# module2.py
def farewell():
return "Goodbye from module2!"
module3.py (inside the subpackage):
# module3.py
def welcome():
return "Welcome from module3!"
script.py:
# script.py
from my_package.module1 import greet
from my_package.module2 import farewell
from my_package.subpackage.module3 import welcome
if __name__ == "__main__":
print(greet())
print(farewell())
print(welcome())
In this example:
- my_package is the main package, containing the special __init__.py file.
- module1.py and module2.py are modules directly inside the package.
- subpackage is a sub-package within my_package and contains its own __init__.py file and module3.py.
- script.py demonstrates how to import functions from modules within the package and sub-package.
Understanding importlib Package
For those seeking a more dynamic approach, the importlib package provides the tools to import modules programmatically. This package is part of the Python standard library and offers a way to import modules using importlib.import_module(). This method is particularly useful when you need to import modules whose names are determined at runtime.
Suppose you have the following directory structure:
dynamic_import/
|– __init__.py
|– module1.py
|– module2.py
|– script.py
module1.py:
# module1.py
def greet():
return "Hello from module1!"
module2.py:
# module2.py
def farewell():
return "Goodbye from module2!"
script.py:
# script.py
import importlib
def import_and_execute(module_name, function_name):
try:
module = importlib.import_module(module_name)
function = getattr(module, function_name)
result = function()
print(result)
except ModuleNotFoundError:
print(f"Module '{module_name}' not found.")
except AttributeError:
print(f"Function '{function_name}' not found in module '{module_name}'.")
if __name__ == "__main__":
import_and_execute("dynamic_import.module1", "greet")
import_and_execute("dynamic_import.module2", "farewell")
In this example:
- The script.py file defines a function import_and_execute that takes a module name and a function name as parameters.
- Using importlib.import_module(), it dynamically imports the module.
- The getattr function is then used to retrieve the function from the imported module.
- The function is executed, and the result is printed.
Using .pth Files
Python’s import system includes a less known feature: .pth files. Placed in the site-packages directory, these files add extra directories to sys.path. Each line in a .pth file specifies a path that Python includes in sys.path. While this method is persistent and doesn’t need code changes, it impacts the entire Python environment globally, which may not be suitable for every scenario.
Best Practices and Pitfalls
To keep your codebase clean and easy to manage, it’s important to import modules wisely from different directories. Avoid messing with sys.path in your production code, use relative imports carefully, and plan your project structure thoughtfully. Watch out for common issues like circular imports and namespace conflicts, as they can make debugging a challenge.
Conclusion
Importing modules from various directories in Python may seem challenging initially, but with the right techniques, it becomes manageable. Whether you’re adjusting sys.path, setting up PYTHONPATH, using relative imports, creating a package, using importlib, or employing .pth files, each method serves a purpose. Consider the advantages and disadvantages of each approach and pick the one that suits your project best. Armed with these tools, you can keep your Python code organized and efficient.
You can also refer our other articles to learn and explore about Python:
Frequently Asked Questions
A1: PYTHONPATH
is an environment variable that allows you to specify additional directories where Python should look for modules and packages. It’s set outside of Python, often in the shell’s configuration or OS environment settings, providing a way to customize the module search path without modifying code.
A2: Modifying sys.path
directly can be a temporary and less maintainable solution. It might lead to code that’s harder to understand and maintain over time. It’s generally recommended to explore alternative approaches like setting up PYTHONPATH
or using relative imports.
A3: Relative imports allow you to import modules based on their location in the directory structure. They work well within a package using dot notation to navigate the hierarchy. However, they may not be suitable for standalone scripts outside of a package.
A4: A package is a directory containing a special file named __init__.py and can include modules and sub-packages. It’s beneficial when you frequently need to import modules from different directories. Structuring your code as a package allows the use of both absolute and relative imports.
A5: The importlib package provides tools to import modules programmatically. It’s useful when you need to import modules dynamically, especially when the module names are determined at runtime.
A6: .pth files, placed in Python’s site-packages directory, add additional directories to sys.path. This method is persistent and doesn’t require code changes. However, it affects the entire Python environment, so use it carefully based on your project’s requirements.